You can see an index of all the posts in this series: go to index.
If you want to review my progress, here are the files from the end of the previous post: first steps.
In the previous post in this series, I started to work on a simple HTML quiz game. One early issue is that currently the answer is shown immediately beneath the question. What can I do about that?
One possible solution is to present the answer on a different HTML page. I could also then show the next question under that. Let me try that. I created a new file called question2.html and saved it in the same folder as my index.html file. At this point you might be wondering why I saved the first file as index.html and not question1.html.
The answer is that if a user navigates to a website without specifying a particular page, then the website will default to serving a page called index.html, if it can find one. In other words, index.html is the home page of the website. While I am developing my site and loading pages locally, it doesn’t matter too much, but if I were to later transfer my files to a web server, it would, so it’s best to get into the habit of following that convention now.
Now I have my question2.html file, I enter the following lines:
<!DOCTYPE html> <html lang="en" dir="ltr"> <head> <meta charset="utf-8"> <title>General Knowledge Quiz - Question 2</title> </head> <body> <h1>General Knowledge Quiz</h1> <p>The answer to question 1 was C. Canberra. Now try question 2:</p> <p>Which of the following are national languages of Switzerland?</p> <ol type="A"> <li>English</li> <li>French</li> <li>German</li> <li>Italian</li> <li>Romansh</li> </ol> </body> </html>
When I save this file and open it in my web browser, I see it looks similar to the first page I created:
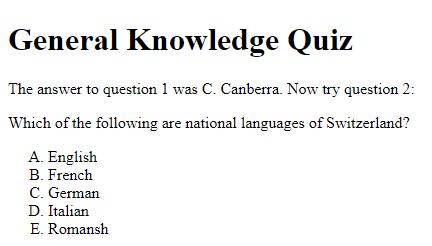
OK so now I have my two questions in separate pages, but how does the user get from my index.html page to my question2.html page?
The HT bit of HTML is short for hypertext. Hypertext is text that has embedded links that allow you to follow references to other documents by clicking on them. So to get to question2.html from index.html I need to create such a link. In index.html I changed the line that currently displays the answer to this:
<p><a href="question2.html">See the correct answer and question 2</a></p>
The change looks like this in the browser:
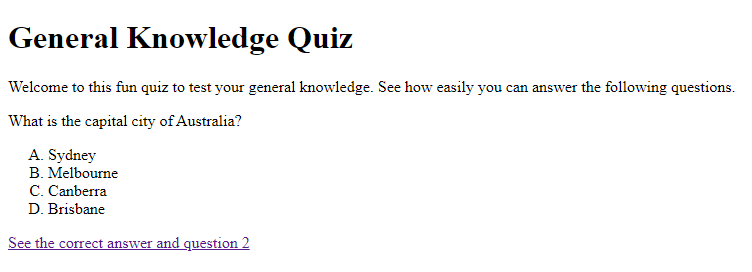
If I click on the link, it will take me to question 2.
If you look at the changed line you can see I’ve introduced a new tag, a. The a stands for anchor. Anchor elements are used to create links between documents or between sections within a document. Any text surrounded by the opening and closing a tags will form the link. in other words, that will be the text a user clicks on to follow the link.
To set the destination for the link I use the href attribute. This must contain a valid URL. URL stands for Uniform Resource Locator and is basically a standard way of referencing web resources. While it’s most common for a URL to reference a web page, it can also reference other types of web resources, e.g. email addresses. Let’s focus on web pages for now though. You’ll commonly see two forms of links to web pages. One is a relative link, which is what I’ve used here. By just referencing the filename, the browser assumes I want a file of that name in the current directory or folder (in a future post I’ll also show you how I can access files in other directories or folders on the same website). However I could also reference a document on a completely different website. For example, if I wanted to link the the Wikipedia home page, I could use:
<a href="https://en.wikipedia.org/wiki/Main_Page">Go to Wikipedia</a>
This uses a fully qualified URL. It’s known as an absolute link. Although the two do look very different, my browser will always convert the relative link to a fully qualified link. For example, if my files were in the root directory at the website http://www.nonexistentwebsite.com, then when the browser sees the link <a href=”question2.html”> it will actually convert that to a link to http://www.noneexistentwebsite.com/question2.html. When I am building my pages, however, it’s best to stick to relative links, so that my pages will continue to work, even if I move them to a different location.
Something else you might have noticed about the link I added is that I put it inside a paragraph element. Why was that?
HTML elements that display content are divided into two types: block-level elements and inline elements.
Block-level elements are the introverts of the HTML world – they want their own space. So, by default, a block-level element will start on a new line and is also followed by a line break. It will also, by default, take up the full width of the content area in the browser. I say by default, because, as you’ll discover in a later post in this series, there are ways I can change this. Some examples of block-level elements that I’ve already used are headings, paragraphs and lists.
Inline elements, by contrast, are the extroverts of the HTML world – they love nothing more than to get up close and friendly with other HTML elements. Inline elements are, by default, presented on the current line, only take up as much space as they need and will not be followed by a line break. Again, this behaviour can be changed, but I’ll come back to that in a future post. The anchor element, as you might have guessed, is an example of an inline element. This means, if I want the link to be shown on it’s own line, I need to place it within a block level element, like p. (Note: unless I change the default behaviour as I mentioned earlier).
Now that I know how to link pages, I can create a third question. But I’ll make this one a picture question. The picture I’ll use is this one:
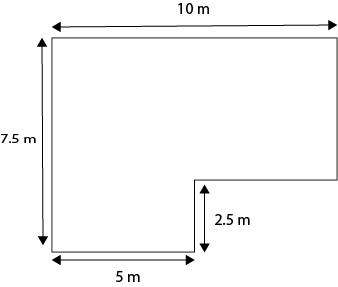
Rather than store my images in the same folder as my HTML files, I’ll create a new folder for them. Inside my quiz folder, I created a new folder called images, then I saved a copy of the image above in that folder.
Now I’ve created a new file in my editor and saved it (in the quiz folder) as question3.html. I entered the following in the file:
<!DOCTYPE html> <html lang="en" dir="ltr"> <head> <meta charset="utf-8"> <title>General Knowledge Quiz - Question 3</title> </head> <body> <h1>General Knowledge Quiz</h1> <p>The answer to question 2 was B. French, C. German, D. Italian and E. Romansh. Now try question 3:</p> <p>What is the total area of the room shown in this plan?</p> <img src="images/html-question3-image.png"> <ol type="A"> <li>10 m<sup>2</sup></li> <li>12.5 m<sup>2</sup></li> <li>50 m<sup>2</sup></li> <li>56.25 m<sup>2</sup></li> <li>62.5 m<sup>2</sup></li> <li>75 m<sup>2</sup></li> </ol> </body> </html>
Most of this should be familiar to you by now, but there are two new elements introduced here. On line 11 I use the image element, which uses the tag img. As you can see img is a self closing tag. The image element displays an image held in an external file. I reference this file with a URL in the src attribute (short for source). Just like the href attribute in anchor elements, the src attribute in image elements will take either an absolute or relative URL. Note that, because the image file is not in the same folder, I need to specify the folder first followed by a forward slash and then the filename.
The second new element is the superscript element, which uses the sup tag. You can see examples of this in each of the list items. This is an inline element that lets the browser know that the text within it is intended to be superscript. There’s a corresponding subscript element that uses the tag sub, which I might use as follows:
<p>The chemical formula for water is h<sub>2</sub>o.</p>
In the browser, the new page looks like this:
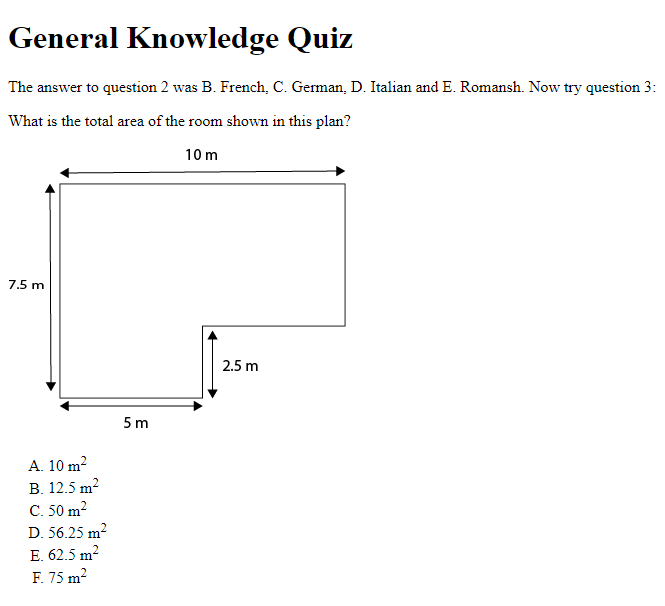
So I’m starting to get a more functional quiz now, but its presentation leaves a lot to be desired – that serif font is not very easy on the eye and some colour and a nicer alignment of the various elements would be nice. In the next post I’ll start to fix this.
In the meantime, here are are some things I’m thinking about. Do you think the image element (img) is a block-level element or an inline element? I’m inclined not to leap to any conclusions based on what I see in question3.html. I’m going to experiment by using img with other elements. For example, I’ll adding an image in the middle of some text inside a paragraph (p) element.
Although I’ve built the page for the third question, it’s not currently linked from the second question. I need to add that link.
Finally, I need to show an answer for question 3. Sop I’ll create a completely new HTML page in my quiz folder, called finalpage.html. On that page I’ll show the answer to question 3 (which is E. 62.5 m2 by the way) and then thank the player for playing. I also want to add a Play again link which takes the player back to the first question of the quiz.
Be First to Comment