You can see an index of all the posts in this series: go to index.
If you want to follow my progress from this point, here are the starter files from the end of the previous post: first steps.
In the first part of this series, I looked at Buzzword, the BASIC program I’ll be converting and I also took my first steps in setting up my development environment.
At the end of the last post, I had a couple of things to consider. The first was to try and get the program to display something other than the message “Hello, World!”, my name for example. Here’s what I came up with:
/******* * Buzzword * * A program to create amusing fake jargon *******/ #include <stdio.h> int main(int argc, const char * argv[]) { printf("Laurence\n"); return 0; }
When I compiled and ran it, this is what I got:

As you can see, printf will display whatever is between the quotation marks.
When I entered git status, I saw this:
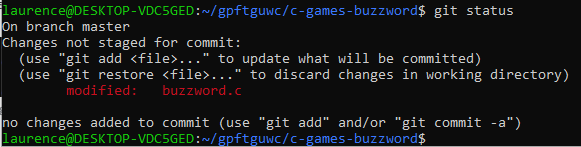
Because I’d made changes since my last commit, Git was indicating that the file had been modified. I entered git diff and I saw this:
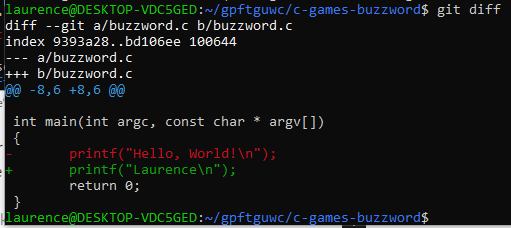
The command git diff shows the changes that have been made to the files in the repository. In this case I’ve changed one line of code. That line is highlighted in green with a +. The previous version of the line with “Hello World!”, is highlighted in red with a -.
To commit that change, I entered git add buzzword.c followed by git commit -m “Change buzzword.c output to my name”. Finally I entered git push origin master as before.
Let’s assume that, having made that change, I slept on it and woke up deciding it was a terrible idea, and I really wanted my program to display “Hello, World!” after all. Here’s where another advantage of using a version control system becomes apparent.
First I closed the file in my editor. Now. in the terminal, I entered git log. I saw this:
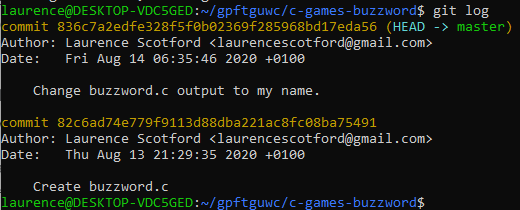
You can see the two commits I’d made here. The bottom one is the first commit I made after creating the file and the top is the one I’d only just made. Notice that the ID for that most recent commit has (HEAD -> master) next to it. That’s indicating that this is the HEAD commit of the master branch – in other words, it’s the commit representing the current state of the repository.
What I want is to remove that commit and make my previous commit the HEAD. To do this I entered git reset --hard HEAD~1
followed by git log. This is what I saw:
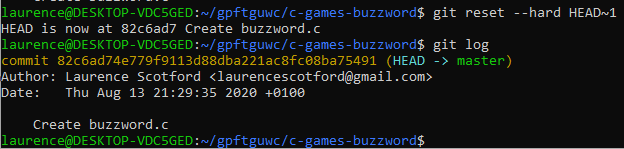
You can see that I now had only the single commit. The git reset command had removed the other commit from the repository. When I use the reset command I have to tell it which commit I want to reset to. I can do this by using the HEAD notation. If HEAD refers to the most recent commit, then HEAD~1 is the commit before that, HEAD~2 is two commits before that and so on. I also entered an option --hard
. This tells Git to not only reset to the earlier commit but also to discard any changes in my local files that were made since the commit I was resetting to. If I didn’t want Git to do this, I could use the --soft
option instead. When I opened the buzzword.c file again in my text editor, I was satisfied to see it was back to the “Hello, World!” version.
There was one last thing to do, which was to push the change to GitHub. In the terminal, I entered git push origin master.
Now I knew how to reset the repository, I could turn my attention to the second of those considerations – what is that strange character combination \n for? I tried moving it to find out. I moved it to the middle of the phrase, as below:
printf("Hello,\n World!");
When I saved and compiled after making this change, I saw this:

You can see that everything after the \n appears on a new line. So that’s what these two characters do.
But why can’t I just hit the enter key in the middle of the message and insert a line break that way? Well I tried it. I deleted the \n and hit the enter key to insert a line break, so that bit of code now looked like this:
printf("Hello, World!");
Now I saved the file and tried to recompile it. The compiler complained and gave me a list of errors.
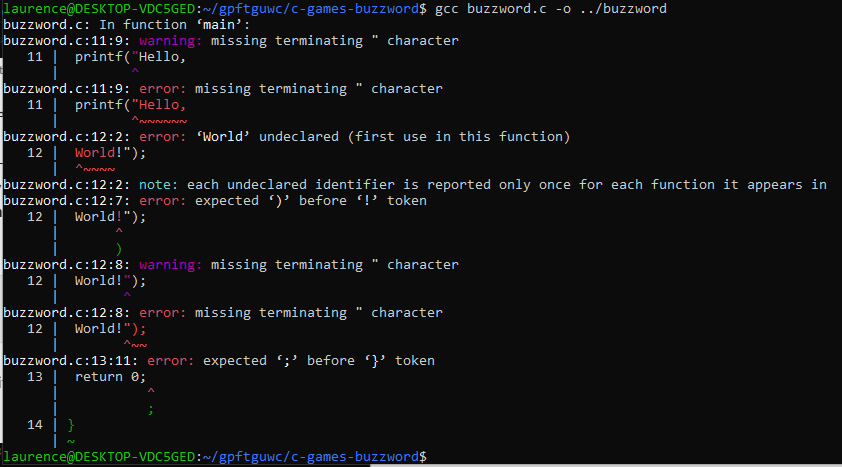
In C it is not permitted to put a physical line break into the middle of quotes like this. In fact there are a whole range of things I can’t insert, like tabs and backspaces for example. Because I might have a legitimate reason for wanting to include new lines or tabs or backspaces, C provides a way to include them by using what are known as escape sequences. These are the backslash character followed by a one letter code. I’ve already shown that \n is used for new line, others include \t for tab and \b for backspace.
You might be wondering what I would do if I wanted to use the backslash character just as a straightforward backslash. Well there’s an escape code for that too – I use a backslash followed by another backslash: \\. A similar situation occurs when I want a double quote mark to appear in my message. If I just include it as it is, the compiler will assume that’s the end of the message and I’ll get an error message again. You’ve probably figured out the solution. Yes, I use yet another escape sequence: \”. Here’s an example of how these are used with printf:
printf("\"When I use a word,\" Humpty Dumpty said, in a rather scornful tone, \"it means just what I choose it to mean - neither more nor less.\"");
Of the six quotation marks used here, the four with a backslash in front of them will be displayed, while the outer two quotation marks indicate to the compiler what I want to display.
You might have noticed that this line of code ends with a semi-colon. A C program is divided into a number of different statements. The semi-colon is how I tell C this is the end of the statement. I must remember to include a semi-colon at the end of every statement. If I don’t, the compiler will complain by showing me error messages until I fix it.
OK it’s time to get started recreating Buzzword. If you refer back to the flowchart in the previous post, you’ll see that the first task is to show an introduction and some instructions. Let’s have a look at the start of the original BASIC program to see how this is done:
10 PRINT TAB(26);"BUZZWORD GENERATOR" 20 PRINT TAB(15);"CREATIVE COMPUTING MORRISTOWN, NEW JERSEY" 30 PRINT:PRINT:PRINT 40 PRINT "THIS PROGRAM PRINTS HIGHLY ACCEPTABLE PHRASES IN" 50 PRINT "'EDUCATOR-SPEAK'THAT YOU CAN WORK INTO REPORTS" 60 PRINT "AND SPEECHES. WHENEVER A QUESTION MARK IS PRINTED," 70 PRINT "TYPE A 'Y' FOR ANOTHER PHRASE OR 'N' TO QUIT." 80 PRINT:PRINT:PRINT "HERE'S THE FIRST PHRASE:"
Note that BASIC uses line numbers as a way of putting statements in order of execution. C doesn’t use line numbers – execution is determined by the order that statements occur in the file.
The command that BASIC uses to show information should look quite familiar, as it is very close to the C equivalent. I had a go at converting this to C and then building and running again. I didn’t worry about the TAB instructions – I’ll show everything left aligned. By the way, when you see a PRINT statement without any quote marks following it, this is the BASIC equivalent of printf(“\n”);, i.e. it just displays a line break.
Here’s what my version looked like:
/******* * Buzzword * * A program to create amusing fake jargon *******/ #include <stdio.h> int main(int argc, const char * argv[]) { printf("Buzzword Generator\nBased on a program from Creative Computing, Morristown, New Jersey\n\n\nThis program prints highly acceptable phrases in 'educator-speak' that you can work into reports and speeches. Whenever a question mark is printed, type a 'Y' for another phrase or 'N' to quit.\n\n\nHere's the first phrase:\n"); return 0; }
Note – that printf statement is just a single line of code without any line breaks – you’re just seeing it with apparent line breaks here because it’s being wrapped to fit in the panel on this page. I could have chosen to use several printf commands rather than just one, that’s fine too, but remember that C will show the output from each of these on a single line. If I want a line break, I need to explicitly put one in using \n.
When I saved, compiled and ran this I saw this:

So that’s the first stage of my program complete. Once it was working correctly for me, I commited a change with a short description, “Change buzzword.c to display instructions for the player.”) and then pushed my changes to GitHub.
In the next part of this series I’ll look at how I can store information in my program.
Be First to Comment