You can see an index of all the posts in this series: go to index.
If you want to review where I got to by this point, here are the starter files from the end of the previous post: variables.
In the previous post in this series, I used variables to store information in my program. I also used arrays to store a collection of variables. At the end of that post I considered a challenge. The first part of challenge was to display the phrase “10 green bottles hanging on the wall”, but to store the number 10 and the word ‘green’ in variables. This is the solution I came up with:
/******* * Buzzword * * A program to create amusing fake jargon *******/ #include <stdio.h> int main(int argc, const char * argv[]) { printf("Buzzword Generator\nBased on a program from Creative Computing, Morristown, New Jersey\n\n\nThis program prints highly acceptable phrases in 'educator-speak' that you can work into reports and speeches. Whenever a question mark is printed, type a 'Y' for another phrase or 'N' to quit.\n\n\nHere's the first phrase:\n"); int number = 10; char colour[] = "green"; printf("\n%i %s bottles hanging on the wall\n", number, colour); return 0; }
When I saved, compiled and ran this, I saw:
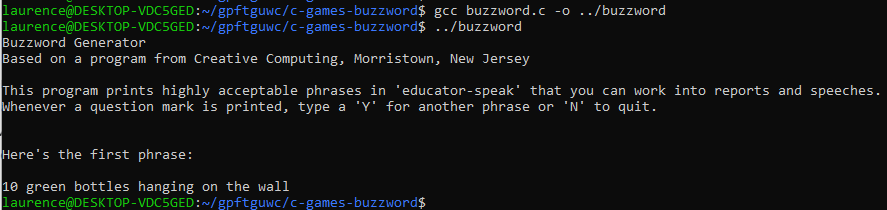
Now that I have a reasonable command of variables and arrays, let’s think how these might be useful to me for storing the list of words.
If I refer back to the original BASIC program, I see code that looks like this:
90 DIM A$(40) 100 FOR I=1 TO 39 : READ A$(I) : NEXT I 200 DATA "ABILITY","BASAL","BEHAVIORAL","CHILD-CENTERED" 210 DATA "DIFFERENTIATED","DISCOVERY","FLEXIBLE","HETEROGENEOUS" 220 DATA "HOMOGENEOUS","MANIPULATIVE","MODULAR","TAVISTOCK" 230 DATA "INDIVIDUALIZED","LEARNING","EVALUATIVE","OBJECTIVE" 240 DATA "COGNITIVE","ENRICHMENT","SCHEDULING","HUMANISTIC" 250 DATA "INTEGRATED","NON-GRADED","TRAINING","VERTICAL AGE" 260 DATA "MOTIVATIONAL","CREATIVE","GROUPING","MODIFICATION" 270 DATA "ACCOUNTABILITY","PROCESS","CORE CURRICULUM","ALGORITHM" 280 DATA "PERFORMANCE","REINFORCEMENT","OPEN CLASSROOM","RESOURCE" 290 DATA "STRUCTURE","FACILITY","ENVIRONMENT"
What’s happening here? Line 90 is actually doing something I’ve already used in C. It is creating an array with 40 elements. In BASIC, I dimension an array when I create it, so DIM is short for dimension.
A$ is the name of the array. String variables in BASIC always end with $. An important thing to note here is that the name of the array in the original BASIC program is just a single letter. This was a common practice at the time this program was written because some BASIC dialects only supported single letter variable names. C suffers no such restriction, so I should always make my variable names as descriptive as possible, while also keeping them succinct. For example, calling a variable s doesn’t really tell me what the variable is for, whereas calling a variable score gives me a good idea what it does.
Lines 200 to 290 contain all the words for the program. At present these are stored as string literals, but the program needs to put them into the array for them to be useful. In BASIC, information can be embedded in the program using the command DATA. When the program needs to make use of this data it can read it into a variable using the command READ. The first READ command will read the first piece of data stored, the second will read the second and so on.
Line 100 is an example of a loop. This repeats this line of code 39 times, and for each time it repeats, it reads another word into an element of the A$ array. When this loop finishes, all the words embedded in the DATA lines will have been stored in the array A$. I’ll revisit loops in a future post.
So in C I’ve seen how to create a single string. For example, I could create a string with the first word, “ability”, with the following line of code:
char word[] = "ability";
But how do I create an array of strings? I could declare an array to hold four words like this:
char * words[4]
You’ll notice this looks very similar to the way I declared a character array. The difference is that there is now an asterisk between the data type and the name of the array. I could now initialise the elements of the array like this:
words[0] = "ability"; words[1] = "basal"; words[2] = "behavioral"; words[3] = "child-centered";
With my previous array examples I’ve been able to declare and initialise the array at the same time. This is also possible with string arrays:
char * words[] = {"ability", "basal", "behavioral", "child-centered"};
So what does the asterisk mean? I’ve already established that in C, when I declare and initialise a simple variable, some memory is set aside to hold the value. When I want to access that memory I use the name of the variable to reference it. Sometimes though, it’s helpful to be able to reference the memory location (or address) of some data directly. I do this using something called a pointer. A pointer is simply a type of variable that holds the address of some data. I use the asterisk to say that I want a pointer rather than a simple variable. So when my line of code above is executed, what I get is something like this:
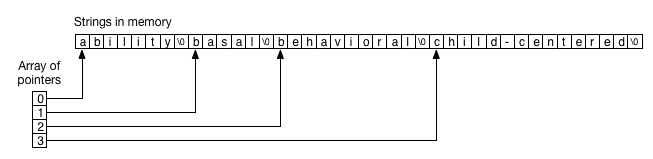
Each of my strings is stored in memory, one after the other. You’ll recall that each string is terminated with a null character (represented by the escape sequence \0). My array then consists of four pointers, and each pointer contains the address in memory of the first character of the associated string. You could say that each element of the array points to its string in memory.
I can use pointers in the same way I used simple variables. So if I wanted to display the second word I could do so this with:
printf("word 2 is %s", words[1]);
OK, let me set up my word lists. Although the original BASIC program placed all the words into a single array, I will place the words into three arrays, for the first adjective words, second adjective words, and noun words respectively. I modified my code so it looked like this (lines 13-15 are new):
/******* * Buzzword * * A program to create amusing fake jargon *******/ #include <stdio.h> int main(int argc, const char * argv[]) { printf("Buzzword Generator\nBased on a program from Creative Computing, Morristown, New Jersey\n\n\nThis program prints highly acceptable phrases in 'educator-speak' that you can work into reports and speeches. Whenever a question mark is printed, type a 'Y' for another phrase or 'N' to quit.\n\n\nHere's the first phrase:\n"); const char * adjectives1[] = {"ability", "basal", "behavioral", "child-centered", "differentiated", "discovery", "flexible", "heterogeneous", "homogenous", "manipulative", "modular", "tavistock", "individualized" }; const char * adjectives2[] = {"learning", "evaluative", "objective", "cognitive", "enrichment", "scheduling", "humanistic", "integrated", "non-graded", "training", "vertical age", "motivational", "creative"}; const char * nouns[] = {"grouping", "modification", "accountability", "process", "core curriculum", "algorithm", "performance", "reinforcement", "open classroom", "resource", "structure", "facility", "environment"}; return 0; }
You’ll notice I’ve added a new keyword, const, to the start of the declaration. This is short for constant, and it tells C that these arrays will not change once they’ve been initialised. When I have variables or arrays that should remain constant, it’s always a good idea to use the const keyword, because the compiler will helpfully show an error message if I try to make changes to those values. This will help me keep errors out of my programs.
In the next part of this series I will create the code to pick words at random from each list and display them. Until then, here’s another challenge I set myself. Display the phrase “flexible cognitive algorithm” by displaying the correct element from each array. Put a new line at the start and end of the phrase and separate each word with a space.
I’ll show my solution the next post.
Be First to Comment